Spring Bean的作用域有哪些?
可以通过scope属性来指定bean的作用域
singleton:默认值。当IOC容器一创建就会创建bean的实例,而且是单例的,每次得到的都是同一个
prototype:原型的。当IOC容器一创建不再实例化该bean,每次调用getBean方法时再实例化该bean,而且每调用一次创建一个对象
request:每次请求实例化一个bean
session:在一次会话中共享一个bean
核心代码
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd">
<!-- ★bean的作用域
可以通过scope属性来指定bean的作用域
-singleton:默认值。当IOC容器一创建就会创建bean的实例,而且是单例的,每次得到的都是同一个
-prototype:原型的。当IOC容器一创建不再实例化该bean,每次调用getBean方法时再实例化该bean,而且每调用一次创建一个对象
-request:每次请求实例化一个bean
-session:在一次会话中共享一个bean
-->
<bean id="book" class="cn.wangzengqiang.interview.atguigu2019.javaee.spring.beans.Book" scope="prototype">
<property name="id" value="8"></property>
<property name="title" value="红高粱"></property>
<property name="author" value="莫言"></property>
<property name="price" value="10.00"></property>
<property name="sales" value="800"></property>
</bean>
</beans>
public class Book {
private Integer id;
private String title;
private String author;
private double price;
private Integer sales;
public Book() {
System.out.println("Book对象被创建了");
}
public Book(Integer id, String title, String author, double price, Integer sales) {
super();
this.id = id;
this.title = title;
this.author = author;
this.price = price;
this.sales = sales;
System.out.println("全参的构造器被调用");
}
public Book(Integer id, String title, String author, double price) {
super();
this.id = id;
this.title = title;
this.author = author;
this.price = price;
System.out.println("不含销量的构造器被调用");
}
public Book(Integer id, String title, String author, Integer sales) {
super();
this.id = id;
this.title = title;
this.author = author;
this.sales = sales;
System.out.println("不含价格的构造器被调用");
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public Integer getSales() {
return sales;
}
public void setSales(Integer sales) {
this.sales = sales;
}
@Override
public String toString() {
return "Book [id=" + id + ", title=" + title + ", author=" + author + ", price=" + price + ", sales=" + sales
+ "]";
}
}
public class SpringTest {
//01.Spring Bean的作用域之间有什么区别
//创建IOC容器对象
ApplicationContext ioc = new ClassPathXmlApplicationContext("beans.xml");
@Test
public void testBook() {
Book book = (Book) ioc.getBean("book");
Book book2 = (Book) ioc.getBean("book");
System.out.println(book == book2);
}
}
/**
* 1.Invalid test class 'cn.wangzengqiang.interview.atguigu2019.javaee.spring.test.SpringTest':
* 1. Method testBook() should be public
* junit测试方法前面应该加个public
* 2.junit方法主类应该是public,否则没有运行按钮
* 3.org.springframework.beans.factory.BeanDefinitionStoreException: IOException parsing XML document from class path resource [beans.xml]; nested exception is java.io.FileNotFoundException: class path resource [beans.xml] cannot be opened because it does not exist
* 在maven工程中,config应该设置为source folder
* 4.非maven工程引入jar包,可以新建一个lib目录,然后设置为source folder,然后添加到类路径
*/
代码结构图
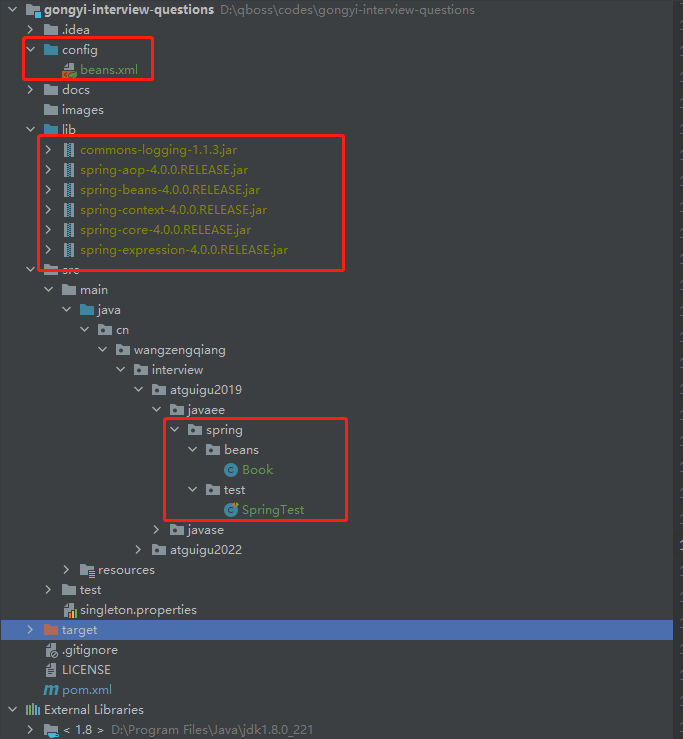
代码地址
代码地址